The Green Padlock Update
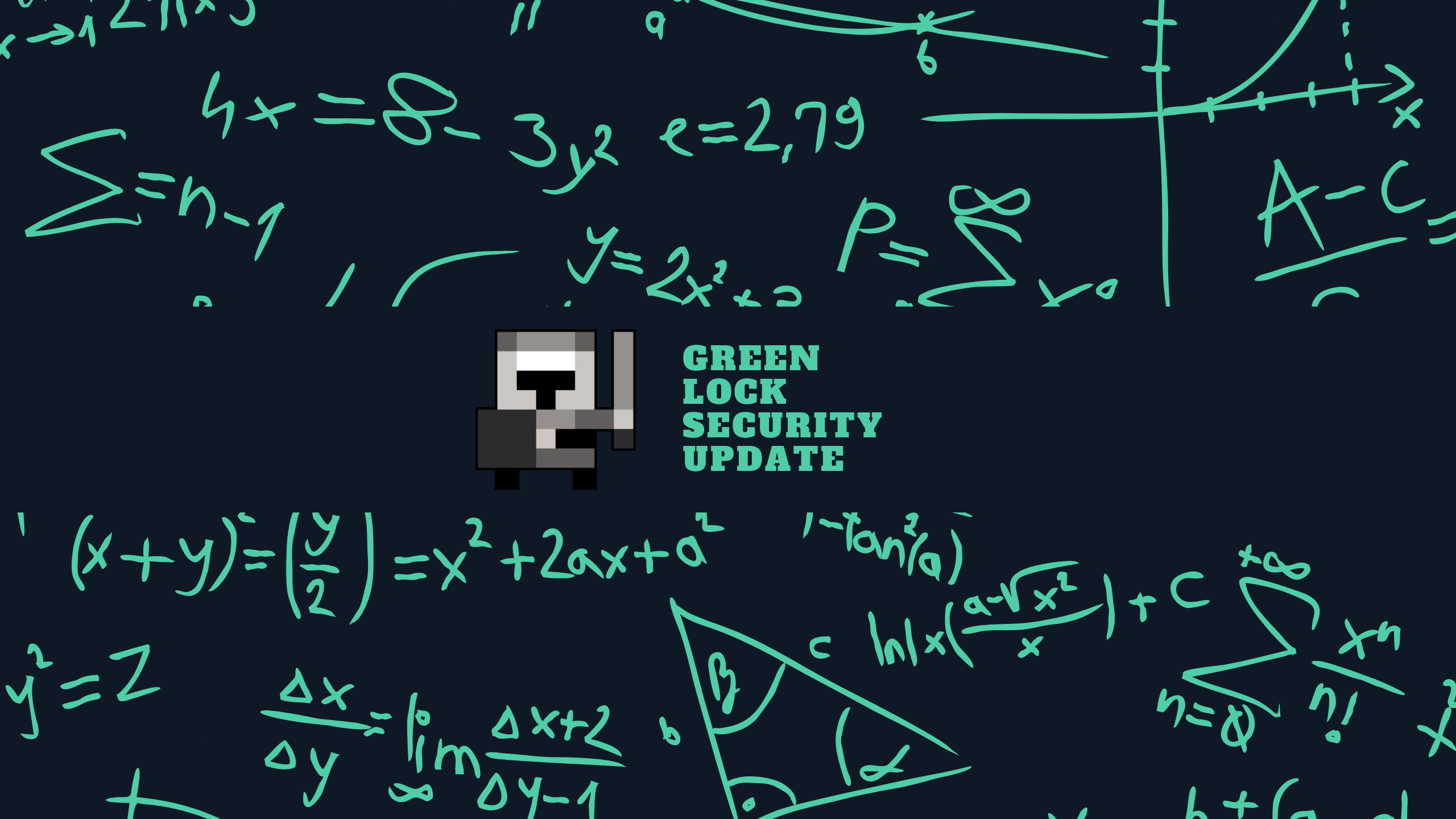
This is not mandatory reading by any means, and is more of a technical writeup than anything else. This is a look at how we implemented https and rate limiting on the backend API. (Finally.)
This article is also put out as a guide or inspiration on how to replicate this on your own server or setup, as we firmly believe that it's much better if everyone is serving over https and DNS to better protect their players, but understand there is zero documentation on how to do this, and the most commonly accepted answer is, "put it on google cloud or aws and let it handle it" isn't really possible for most peoples budgets.
The Problem
- An exposed http server in 2021 == super not good.
- The webserver was built by people screwing around with friends, before https was a super enforced norm and does not just "take a cert".
- The default api uses a combination of deflate and gzip headers, which needs to be accounted for.
The Solution
We went tunneling. We implemented cloudflared - a lightweight proxy daemon.
- Block off all requests from origin and make sure they are proxied through cloudflare.
- Setup IPTables to enable localhost loopback so cloudflared can access the
8080
port but nobody else.
Things To Consider
- You must block off origin requests to the http server, or this whole thing is useless.
- When attempting to reverse proxy through nginx without cloudflared, headers and packets dropped frequently no matter the tuning.
- We want to only allow https traffic and we want to enable HSTS for added security.
Benefits Of The New Setup
- Upgrade all traffic to TLS 1.3 and HTTP/2 (Enforce Web Standards without rewriting the whole codebase)
- Deny ips by ASN. (We don't allow any DigitalOcean or Linode IPs for example.)
- Top of the line rate limiting for login and other secure endpoints.
- Implement caching for commonly requested routes.
Things To Know
1) Brottli compression will break your api over https.
- Most CDN/WAFs including cloudflare will apply what is called "Brottli" compression when serving traffic over https. There should be a setting in your CDN to disable this.
2) Avoid double nested subdomains.
- When choosing a subdomain avoid double nested subdomains such as
valor.proxy.valorserver.com
and instead usevalor-proxy.valorserver.com
. - This is a rather obscure issue, but when using a double nested subdomain on cloudflare, it will generate an
SSL cipher issue
without an Enterprise plan. (Took seven hours to figure out. Yay! 🎉)
Client Changes To Support DNS
The default AS3 client is not setup to support DNS, and only uses hardcoded IPs & Ports. This is a modified function for creating the DNS URL.
public static function buildURIDNS(hostProtocol:String,
hostDomain:String):String {
return URI_PATTERN_DNS.replace(URI_HOST_PROTOCOL, hostProtocol)
.replace(URI_HOST_DOMAIN, hostDomain);
}
IPTable Rules
These are the rules that allow local loopback, and block off the 8080
port from outsiders, so they can't just proxy the origin server.
sudo iptables -A INPUT -i lo -j ACCEPT
sudo iptables -A INPUT -m conntrack --ctstate RELATED,ESTABLISHED -j ACCEPT
#block off port 8080
iptables -A INPUT -p tcp -m multiport --dports 8080 -j DROP